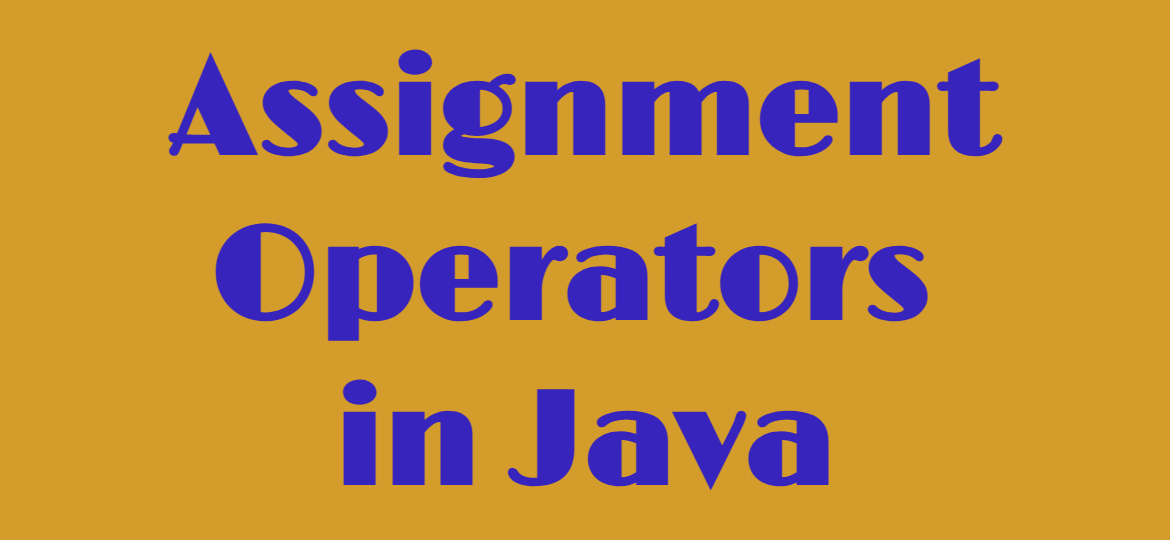
6 Essential Assignment Operators in Java for Smarter and More Efficient Coding
- Assignment Operators in Java
- What are Assignment Operators in Java?
- Why Are Assignment Operators Important?
- Basic Assignment Operator (=)
- Compound Assignment Operators in Java
- Common Errors and Debugging Tips
- Code Optimization with Assignment Operators
- Assignment Operators with Different Data Types
- Comparison with Other Languages
- Conclusion
- Frequently Asked Questions (FAQs)
Assignment Operators in Java
Assignment operators in Java are used to assign values to variables. They provide a convenient way to perform operations while assigning values. Java provides several types of assignment operators that can be used to simplify and enhance code efficiency.
What are Assignment Operators in Java?
Assignment operators are special symbols that assign values to variables in Java. These operators help perform arithmetic operations and store the result in the same variable.
Why Are Assignment Operators Important?
- They make code more readable and concise.
- They improve performance by reducing redundant operations.
- They are widely used in loops, expressions, and calculations.
Basic Assignment Operator (=
)
The simplest assignment operator in Java is =
, which assigns the value on the right-hand side to the variable on the left-hand side.
Example:
public class AssignmentExample { public static void main(String[] args) { int a = 10; // Assigning value 10 to variable a System.out.println("Value of a: " + a); } }
Output:
Value of a: 10
Compound Assignment Operators in Java
Java provides several compound assignment operators that perform an operation and assignment in a single step, making code shorter and more efficient.
Addition Assignment (+=
)
This operator adds the right-hand value to the left-hand variable and assigns the result to the variable.
Example:
public class AdditionAssignment { public static void main(String[] args) { int b = 5; b += 3; // Equivalent to b = b + 3; System.out.println("Value of b: " + b); } }
Output:
Value of b: 8
Subtraction Assignment (-=
)
This operator subtracts the right-hand value from the left-hand variable and assigns the result.
Example:
public class SubtractionAssignment { public static void main(String[] args) { int c = 10; c -= 4; // Equivalent to c = c - 4; System.out.println("Value of c: " + c); } }
Output:
Value of c: 6
Multiplication Assignment (*=
)
This operator multiplies the left-hand variable by the right-hand value and assigns the result.
Example:
public class MultiplicationAssignment { public static void main(String[] args) { int d = 7; d *= 2; // Equivalent to d = d * 2; System.out.println("Value of d: " + d); } }
Output:
Value of d: 14
Division Assignment (/=
)
This operator divides the left-hand variable by the right-hand value and assigns the quotient.
Example:
public class DivisionAssignment { public static void main(String[] args) { int e = 20; e /= 5; // Equivalent to e = e / 5; System.out.println("Value of e: " + e); } }
Output:
Value of e: 4
Modulus Assignment (%=
)
This operator assigns the remainder of the division to the variable.
Example:
public class ModulusAssignment { public static void main(String[] args) { int f = 15; f %= 4; // Equivalent to f = f % 4; System.out.println("Value of f: " + f); } }
Output:
Value of f: 3
Common Errors and Debugging Tips
Avoiding Mistakes with Assignment Operators
- Using
= instead of
== in conditions: A common mistake is using
=
instead of==
in conditional statements.
int x = 5; if (x = 10) { // Compilation error: incompatible types System.out.println("X is 10"); }
- Handling division assignment carefully: Avoid division by zero errors when using
/=
. - Using parentheses for clarity: When mixing multiple operators, always use parentheses to ensure clarity and prevent unintended results.
Code Optimization with Assignment Operators
Enhancing Performance and Readability
- Using assignment operators in loops reduces redundant operations and improves efficiency.
for (int i = 0; i < 10; i += 2) { System.out.println(i); }
- Optimizing memory usage: Instead of creating new variables for calculations, modify existing ones where possible.
- Writing clean and concise code: Using assignment operators makes code more readable and maintainable.
Assignment Operators with Different Data Types
String, Floating-Point, and Character Assignments
- Strings:
+=
can be used for concatenation. - Floating Points: Works the same as with integers but with precision.
- Characters: Can be incremented using
+=
since characters are stored as ASCII values.
Example:
public class AssignmentWithTypes { public static void main(String[] args) { String text = "Hello"; text += " World!"; System.out.println(text); double num = 5.5; num *= 2; System.out.println(num); char letter = 'A'; letter += 1; // ASCII increment System.out.println(letter); } }
Output:
Hello World! 11.0 B
Comparison with Other Languages
- Python: Uses
+=
,-=
, etc., similarly but can be used with lists and tuples. - C++: Works the same as Java but also supports pointer assignment.
- JavaScript: Similar to Java, used for numbers and strings.
Conclusion
Assignment operators in Java provide a convenient way to perform operations while assigning values to variables. Understanding these operators allows for writing more efficient and concise code. By mastering both basic and compound assignment operators, developers can improve their coding skills and reduce redundancy in Java programs.
Frequently Asked Questions (FAQs)
What is the difference between =
and ==
in Java?
=
is the assignment operator, while==
is the equality comparison operator.
How do assignment operators improve performance?
- They reduce redundant calculations and optimize memory usage.
Can assignment operators be used with strings?
- Yes, the
+=
operator is commonly used for string concatenation in Java.
By implementing these best practices, developers can write more efficient and error-free Java programs!
If you like this post, please share and comment. Visit my blog for more articles.
About the author : Mohit Jain
My name is Mohit Jain. I hold a masters’ degree in computers, and I am currently working in an MNC as a Java Developer. I have almost six years of work experience. I have used technologies like Java 8, Hibernate, Spring Framework and Spring Boot, MySQL and PostgreSQL along with AWS (mostly) during my tenure. I have earned multiple certifications as well like Oracle Certified Associate (OCA – Java 8), AWS Cloud Practitioner, and AWS Solution Architect, etc. I like sharing my knowledge with others, so I started this blog.