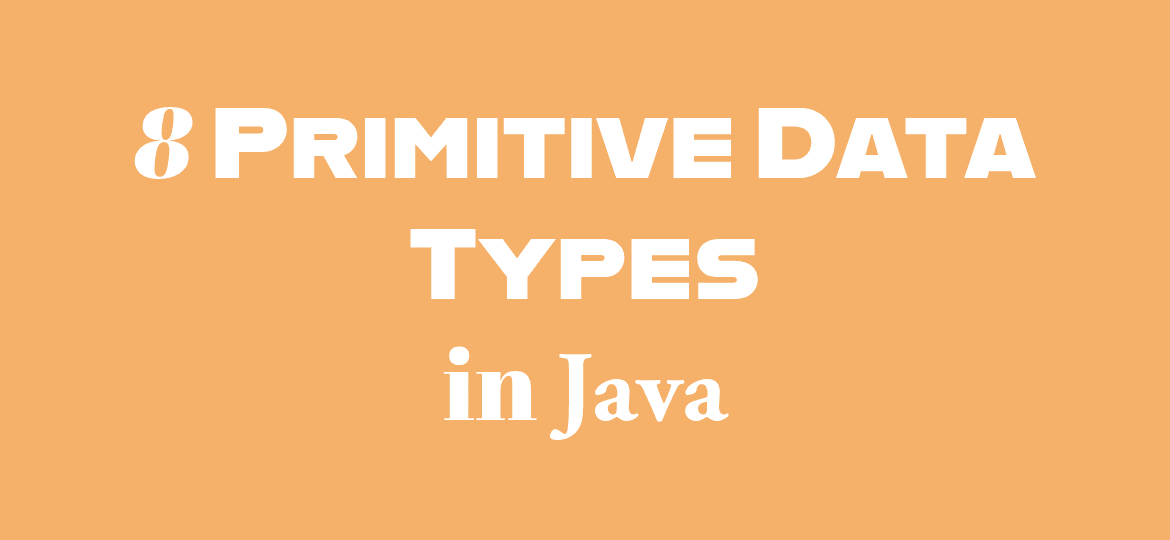
8 Primitive Data Types in Java: A Complete Guide with Examples
What Are Primitive Data Types?
Java is a statically typed language, meaning every variable must have a specific type. The fundamental building blocks of data handling in Java are primitive data types. These types represent simple values and are not objects, making them highly efficient in terms of memory and performance.
There are eight primitive data types in Java, categorized based on the kind of values they store. These are:
- byte
- short
- int
- long
- float
- double
- char
- boolean
Unlike reference types (objects), primitive data types in Java store actual values rather than references to objects. They are stored in stack memory, making them faster and more memory-efficient.
Integer Data Types
Integer data types store whole numbers (without decimal points). Java provides four integer types with different memory sizes.
byte (1 byte = 8 bits)
- Stores small integer values (-128 to 127).
- Useful for saving memory in large data arrays.
- Default value:
0
Example:
public class ByteExample { public static void main(String[] args) { byte smallNumber = 100; System.out.println("Byte value: " + smallNumber); } }
short (2 bytes = 16 bits)
- Stores integers in the range -32,768 to 32,767.
- Less commonly used than
int
. - Default value:
0
Example:
public class ShortExample { public static void main(String[] args) { short value = 15000; System.out.println("Short value: " + value); } }
int (4 bytes = 32 bits)
- The most commonly used integer type.
- Stores values from -2,147,483,648 to 2,147,483,647.
- Default value:
0
Example:
public class IntExample { public static void main(String[] args) { int number = 100000; System.out.println("Integer value: " + number); } }
long (8 bytes = 64 bits)
- Stores very large integer values (-9 quintillion to 9 quintillion).
- Must be followed by ‘L’ when assigning values.
- Default value:
0L
Example:
public class LongExample { public static void main(String[] args) { long bigNumber = 9000000000L; System.out.println("Long value: " + bigNumber); } }
Floating-Point Data Types
Used for numbers with decimal points (fractions).
float (4 bytes = 32 bits)
- Stores up to 7 decimal digits.
- Must be followed by ‘F’ in assignments.
- Default value:
0.0f
Example:
public class FloatExample { public static void main(String[] args) { float price = 10.99F; System.out.println("Float value: " + price); } }
double (8 bytes = 64 bits)
- Stores up to 15-16 decimal digits.
- More precise than
float
, making it the default for decimal values. - Default value:
0.0d
Example:
public class DoubleExample { public static void main(String[] args) { double distance = 12345.6789; System.out.println("Double value: " + distance); } }
Character Data Type
char (2 bytes = 16 bits)
- Stores a single character enclosed in single quotes (‘ ‘).
- Uses Unicode, allowing it to store any character from different languages.
- Default value:
'\u0000'
(null character).
Example:
public class CharExample { public static void main(String[] args) { char letter = 'A'; System.out.println("Character value: " + letter); } }
Boolean Data Type
boolean (1 bit)
- Stores only true or false values.
- Default value:
false
.
Example:
public class BooleanExample { public static void main(String[] args) { boolean isJavaFun = true; System.out.println("Boolean value: " + isJavaFun); } }
Why Use Primitive Data Types in Java?
- Efficiency – Primitive types are stored directly in memory, making them faster than objects.
- Memory Optimization – They consume less memory compared to reference types.
- Simplicity – They provide straightforward value storage without complex object creation.
Type Conversion in Primitive Data Types
Java allows implicit (widening) and explicit (narrowing) conversions between primitive types.
Implicit Conversion (Widening Casting)
Smaller types automatically convert to larger types.
Example:
public class WideningExample { public static void main(String[] args) { int num = 100; double convertedNum = num; // int to double (automatic) System.out.println("Converted double: " + convertedNum); } }
Explicit Conversion (Narrowing Casting)
Larger types need explicit conversion to smaller types.
public class NarrowingExample { public static void main(String[] args) { double num = 99.99; int convertedNum = (int) num; // double to int (manual) System.out.println("Converted int: " + convertedNum); } }
Conclusion
The primitive data types in Java provide the foundation for all data manipulation in the language. Understanding how they work, their memory sizes, and default values is crucial for writing efficient Java programs. Since primitive types store actual values instead of references, they are much faster and memory-efficient compared to objects.
By mastering these types, you’ll build a strong foundation for more complex Java programming concepts like arrays, collections, and object-oriented programming.
If you like this article, please comment and share, and visit the blog to read more about Java.
About the author : Mohit Jain
I have more than five years of work experience as a Java Developer. I am passionate about teaching and learning new technologies. I specialize in Java 8, Hibernate, Spring Framework, Spring Boot, and databases like MySQL, and Oracle. I like to share my knowledge with others in the form of articles.